I was working on some ASP.NET Core MVC demo code for an article and needed to display/edit a DateTime
property value. This feels like something I've done about 2 zillion times in my career as a dev but I just couldn't get it to work.
Here's what my code looked like in my Edit.cshtml
file:
<div class="form-group mb-3">
<label asp-for="BirthDate" class="control-label"></label>
<input asp-for="BirthDate" class="form-control" />
<span asp-validation-for="BirthDate" class="text-danger"></span>
</div>
Looks completely normal and basic. And yet, here's what I was getting in the browser.
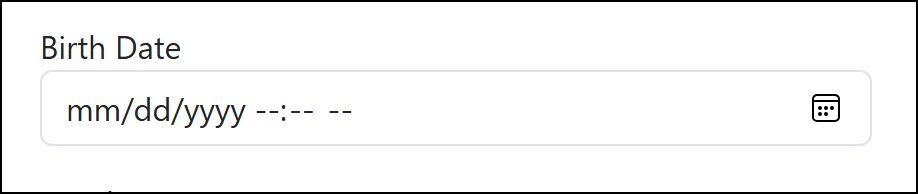
Instead of seeing the value — and there's definitely a value — I was getting mm/dd/yyyy --:-- --
.
Solution
I was surprised by the solution because I would have figured that ASP.NET would generate the right HTML code. Instead, ASP.NET seems to default to a field type of "date" and that was why I was getting a date picker.
To make it work the way I wanted, all I needed to do was add an attribute: type="text"
. Here's the updated code:
<div class="form-group mb-3">
<label asp-for="BirthDate" class="control-label"></label>
<input type="text" asp-for="BirthDate" class="form-control" />
<span asp-validation-for="BirthDate" class="text-danger"></span>
</div>
And when I ran the application, now I see the formatted date in the text box.
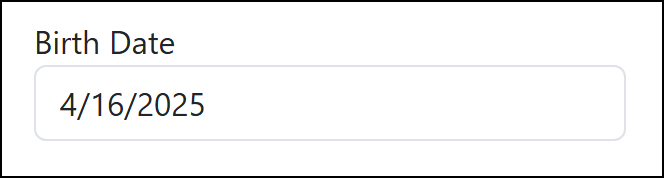
If you want the source code, it's available at https://github.com/benday-inc/demo-aspnet-template.
I hope this helps.