We help teams get productive, cut waste, and deliver great software.
Looking for help with Scrum, Azure DevOps, Team Foundation Server (TFS), software development or technical leadership? We can help.
Drop us a lineOur Services
Want to be more Agile? Looking for an Agile transformation? Need Scrum training or someone to help your Scrum teams improve their delivery game?
We can help. We offer a variety of Scrum courses. We also can embed with your teams to help them develop their Agile & Scrum “muscle memory”.
Ever get the feeling that one or more of your teams just isn’t “clicking” and kind of needs a therapist? Do you suspect there’s a general malaise in your development organization? Do you need someone to work with your executives to improve software delivery practices at the organization level? We can help with that, too.
Whether you’re using Azure DevOps or GitHub, we can help your team collaborate more effectively, streamline your development process, and deliver great software faster.
Both platforms offer powerful DevOps automation capabilities including CI/CD, automated testing, release management, and deep integration with Microsoft Azure. Azure DevOps provides robust tools for planning, tracking, and managing code across teams, while GitHub brings modern, cloud-native workflows with GitHub Actions, Codespaces, and GitHub Copilot to boost productivity.
We’ve helped teams migrate from on-premises TFS to Azure DevOps Services, convert from TFVC to Git, adopt GitHub Enterprise, and build reliable deployment pipelines. If you’re ready to modernize your development process or bridge the gap between platforms, we can help with that, too.
Software development doesn’t have to be painful and software testing doesn’t have to be a magical black box.
We can help your developers learn and adopt the skills to help them to build high-quality, maintainable code. We can help your QA testers plan and manage their work to help ensure quality in your final product. We can help your managers figure out what's going on.
We’ll help everyone learn to work together so that quality becomes something you think about every day rather than something you think about at the last second.
Not sure how to best use Microsoft Azure? We can help with that, too.
Recent Blog Posts
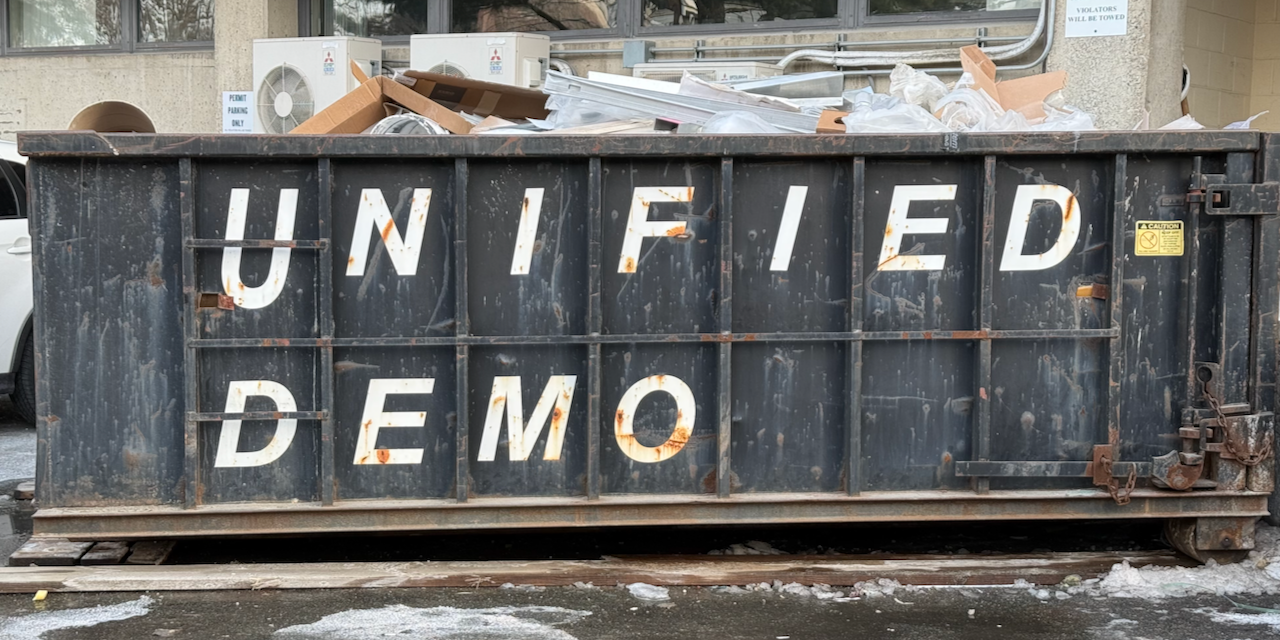